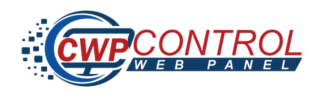
This page is used to test the proper operation of the HTTP server after it has been installed. If you can read this page it means that the HTTP server installed at this site is working properly.
If you are a member of the general public:
The fact that you are seeing this page indicates that the website you just visited is either experiencing problems or is undergoing routine maintenance.
If you would like to let the administrators of this website know that you've seen this page instead of the page you expected, you should send them e-mail. In general, mail sent to the name "webmaster" and directed to the website's domain should reach the appropriate person.
For example, if you experienced problems while visiting www.example.com, you should send e-mail to "[email protected]".
If you are the website administrator:
You may now add content to the directory /home/USERNAME/public_html. Note that until you do so, people visiting your website will see this page and not your content. To prevent this page from ever being used, delete index.html in /public_html.
You are free to use the images below on Linux powered HTTP servers. Thanks for using CentOS-WebPanel!